In the past I wrote a post how to migrate Windows service to Azure using Azure WebJobs. Another option for migration of Windows service to Azure is to use Azure Functions.
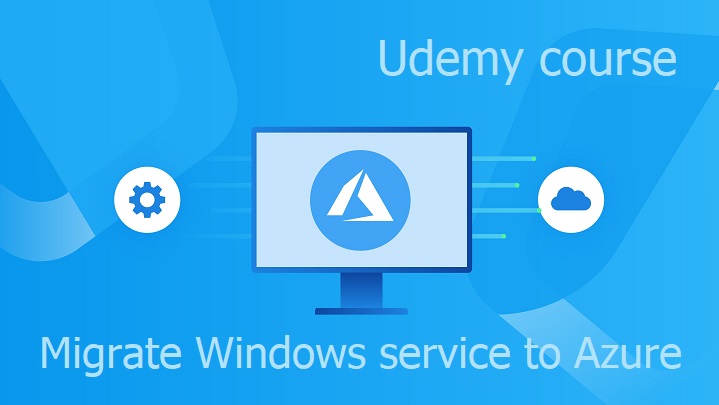
Windows service I want to migrate to Azure acts as runtime for execution of scheduled jobs using Timer
, which run every 60 seconds to execute scheduled jobs. JobSchedulerService
execute JobExecutor.Execute()
method every 60 seconds as visible in source code of Windows service:
public partial class JobSchedulerService : ServiceBase
{
private Timer timer;
public JobSchedulerService()
{
InitializeComponent();
}
protected override void OnStart(string[] args)
{
timer = new Timer();
timer.Elapsed += OnTimer;
timer.Interval = 60 * 1000;
timer.Start();
}
protected override void OnStop()
{
timer.Stop();
}
private void OnTimer(object sender, ElapsedEventArgs e)
{
JobExecutor.Execute();
}
}
To convert Windows service to Azure Function, add new project to Visual Studio solution:
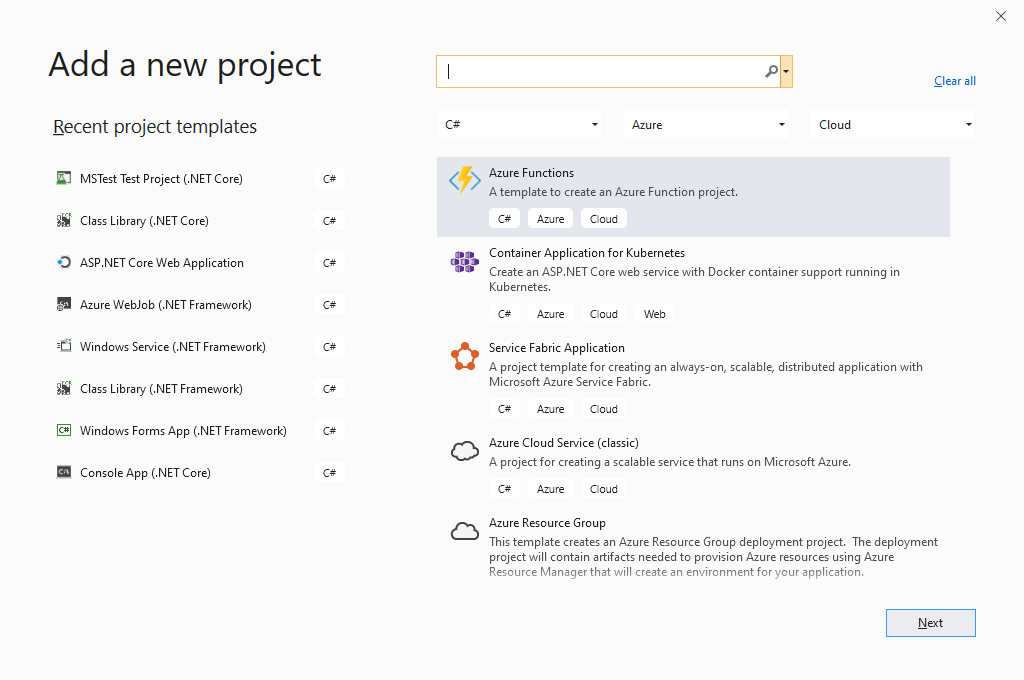
Select Azure Functions v1 (.NET Framework) as version, because Windows service is build on .NET Framework 4.7.2. Then select Timer trigger, none Storage Account and enter schedule “0 * * * * *”, which is CRON expression for triggering run every 60 seconds.
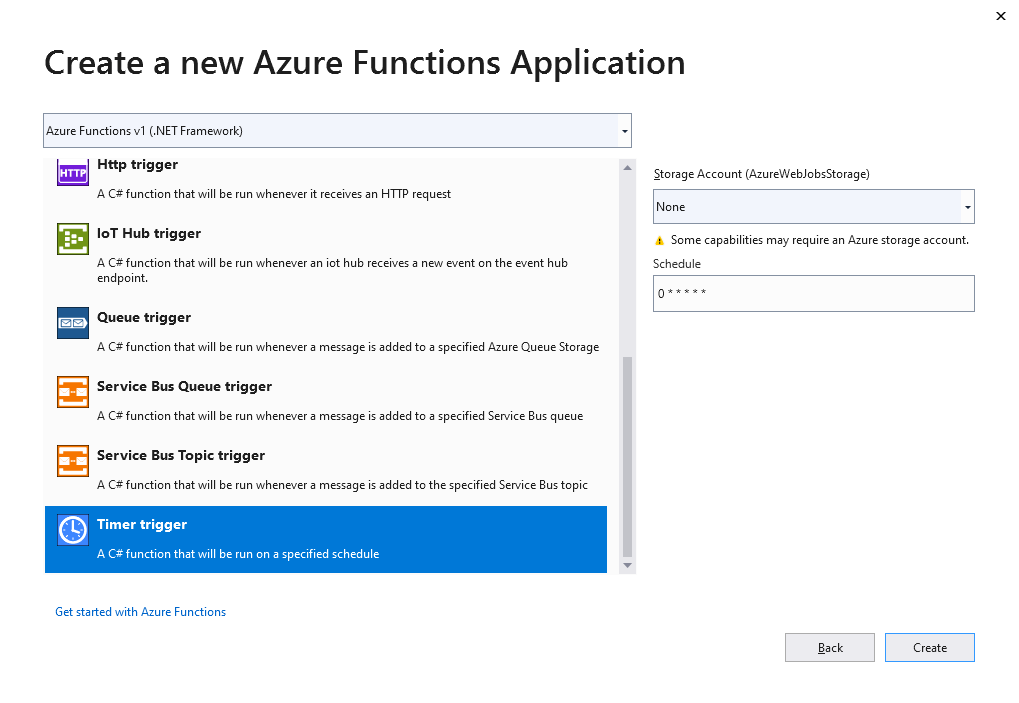
Code changes in function are simple, only call of JobExecutor.Execute()
is added into Run
method:
public static class JobSchedulerFunction
{
[FunctionName("JobSchedulerFunction")]
public static void Run([TimerTrigger("0 * * * * *")]TimerInfo timer, TraceWriter log)
{
JobExecutor.Execute();
}
}
Next step is to create Azure resources to run Azure Function using Azure CLI:
# Before running this script check if Application Insights extension is installed:
# az extension show --name application-insights
# If not installed, install it by:
# az extension add --name application-insights
az group create --name WinServiceToAzureTest --location westeurope
az storage account create --resource-group WinServiceToAzureTest --name winservicetoazuretest --sku Standard_LRS --kind StorageV2
az monitor app-insights component create --resource-group WinServiceToAzureTest --app WinServiceToAzureTest --location westeurope --kind web
az functionapp create --name WinServiceToAzureTest --resource-group WinServiceToAzureTest --storage-account winservicetoazuretest --app-insights WinServiceToAzureTest --consumption-plan-location westeurope --runtime dotnet
az functionapp config appsettings set --name WinServiceToAzureTest --resource-group WinServiceToAzureTest --settings "FUNCTIONS_EXTENSION_VERSION=~1"
After finishing of run is created new Resource Group, Storage Account, Application Insights and finally Function App. Important part is here to set Azure Functions runtime to version 1 using application setting FUNCTIONS_EXTENSION_VERSION
.
Deployment of Azure Functions to Azure can be done by combination of PowerShell and Azure CLI:
Compress-Archive -Path .\bin\Release\net472\* -DestinationPath WindowsServiceToAzure.Job.Function.zip
az functionapp deployment source config-zip --resource-group WinServiceToAzureTest --name WinServiceToAzureTest --src .\WindowsServiceToAzure.Job.Function.zip
After deployment is Azure Function running, which is visible on Function App dashboard:
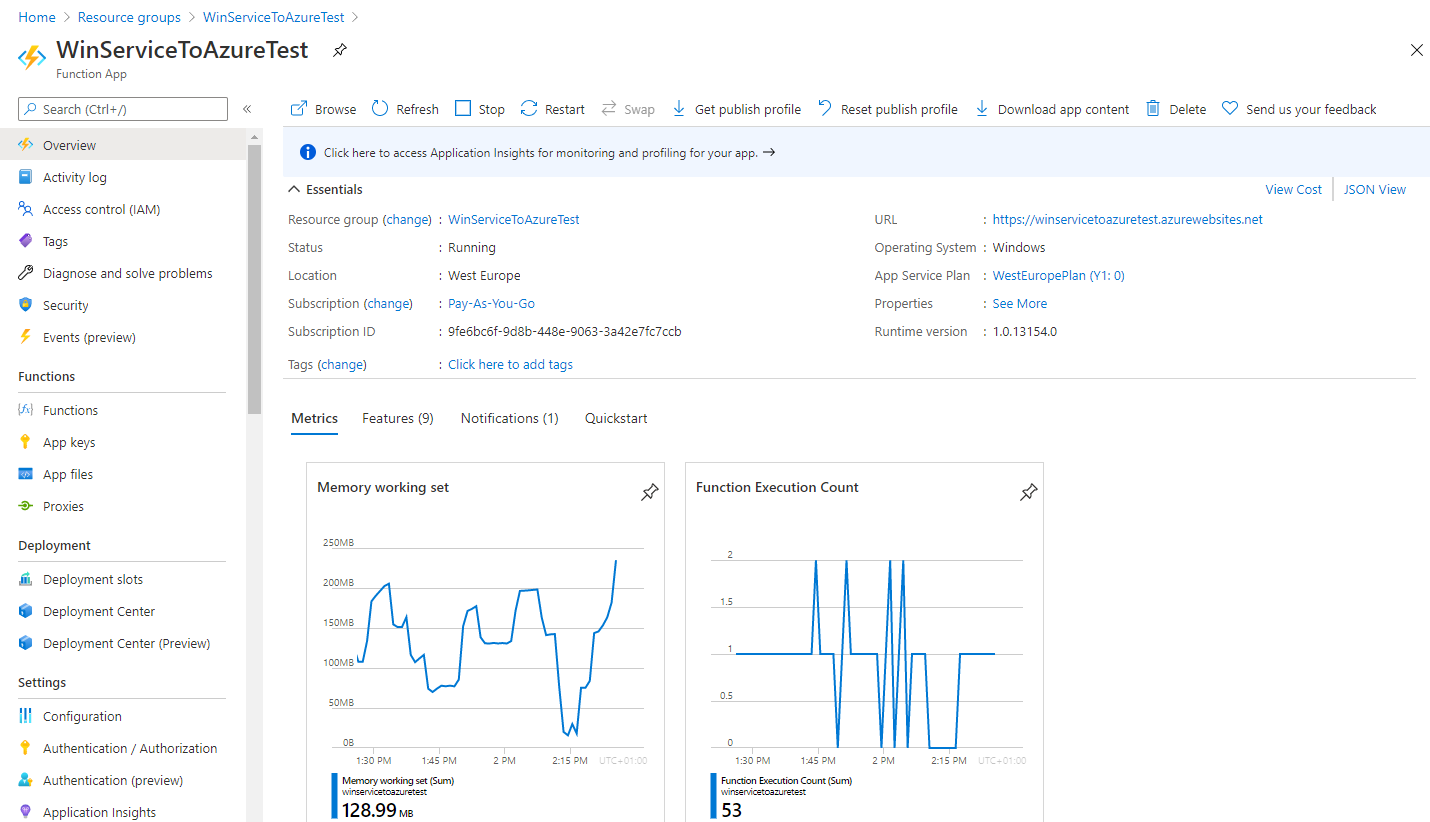
Azure Function integration diagram displays function input and outputs:
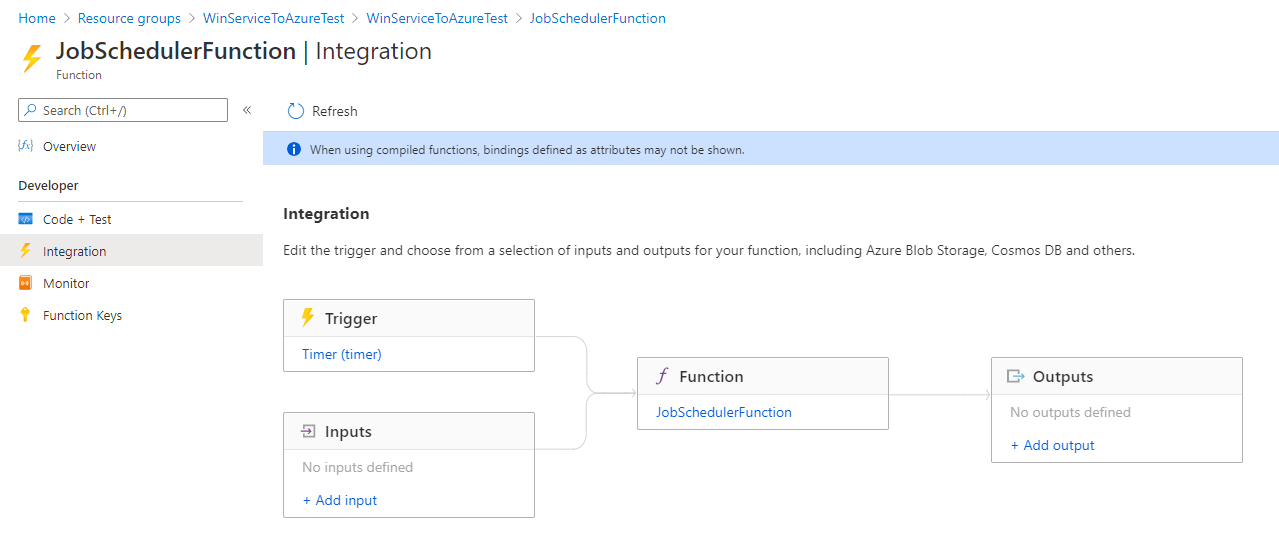
Detailed logs about the activity of Azure Function is visible in monitor:
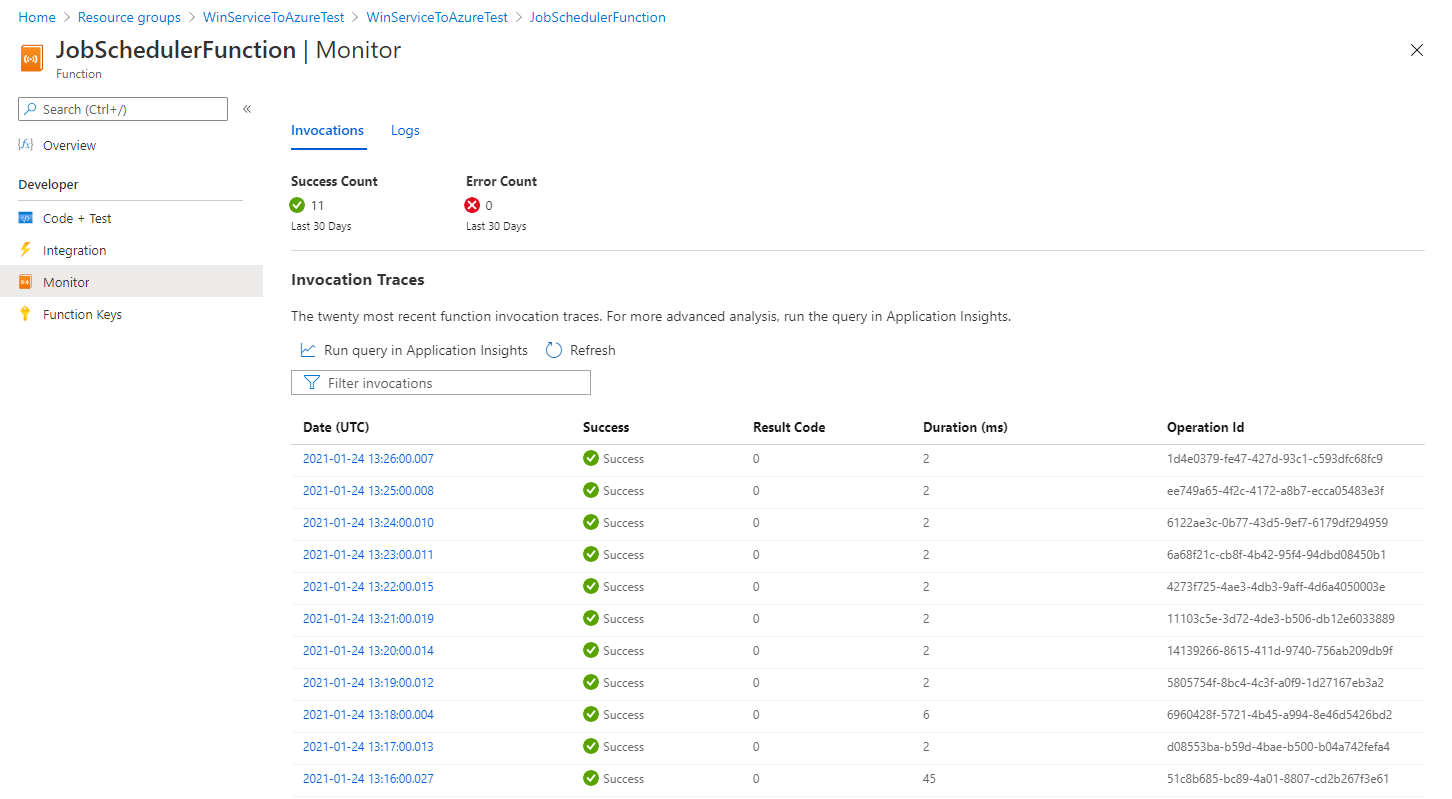
Cleanup of used Azure resources can be done using Azure CLI:
az group delete --name WinServiceToAzureTest --yes -y
If you are interested in Windows services and how to migrate them to Azure, take my Udemy course Migrate Windows service to Azure.